ttk module --> by myCODEnotein
Importing ttk module from tkinter (required)
All the widgets here require ttk module to be imported. This needs to be done explicitly in the code
from tkinter import ttk
Click the link for : tkinter notes
Combobox in tkinter
Creating the combobox
Combobox = Entry Widget + OptionMenu Widget
myCombo = ttk.Combobox(master,**options)
'''
To set values in myCombo
give keyword argument values
which takes a list/tuple as an argument.
'''
myCombo = ttk.Combobox(master,values=[1,2,3,4])
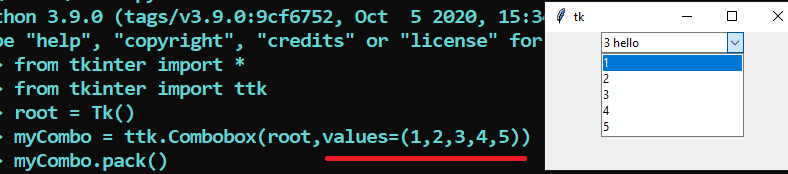
Setting/Getting the value of combobox
myCombo.set(value) # sets the value of combobox
myCombo.get() # returns the value of combobox.
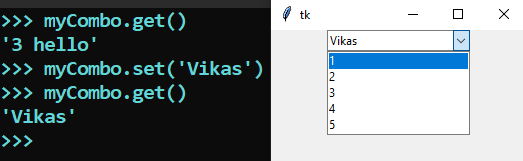
Virtual Event : Runs whenever user selects value from combobox
If you want to run a function whenever user selects value from combobox
then you can bind the combobox with an event (known as virtual event)
by the following command.
myCombo.bind("<<ComboboxSelected>>",function)
# example shown below
Progressbar in tkinter
myProgressbar = ttk.Progressbar(master,**options)
**options include:
orient : "horizontal" or "vertical"
length : what is the length (an integer value)
mode : "determinate" or "indeterminate"
# Just gives different forms of progressbar
value : value is an integer less than length
which determines how
much the progressbar is filled.
and many more
Example
myProgessbar = ttk.Progressbar(master
,length=100,mode="determinate",orient="horizontal")
myProgessbar.pack()
# The image of the following code is below:
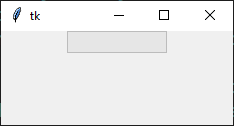
Updating the progessbar
Now to move the progressbar you need to change the value of the progressbar by the following code.
myProgressbar["value"] = someIntegerValue
# someIntegerValue<=length
#For example
myProgressbar["value"] = 50
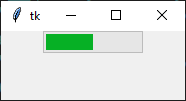
Notebook widget in tkinter
Syntax of Notebook Widget
myNotebook = ttk.Notebook(master,**options)
# myNotebook.pack/grid/place()
Creating a Notebook Widget Example
from tkinter import *
from tkinter import ttk
root = Tk()
myNotebook = ttk.Notebook(root)
myNotebook.pack(expand=True,fill=BOTH)
frame1 = Frame(myNotebook,bg="blue")
# Now you need to add this to the myNotebook instance
myNotebook.add(frame1,text="Frame1")
# Here frame1 can be used as tab_id (explained below)
frame2 = Frame(myNotebook,bg="red")
myNotebook.add(frame2,text="Frame2")
root.mainloop()
Add Method of Notebook Widget
myNotebook.add(widget,**options)
'''Add the tab to the Notebook widget
as shown in the above example.'''
**options
state, sticky, padding, text, image, compound, underline
Id of a tab in Notebook widget
tab_id with index
The tab is given index by tkinter which is similar to that in a list.
Hence the first tab which you add is of index = 0
,second tab if of index=1 and so on.
The currently selected tab can be used by a special index "current" .
tab_id with name
you can also give the name of widget in place of tab_id.
The name of widget is that variable
which stores the instance of the widget.
So in above example
frame1 and frame2 are the names of the widget.
tab_id at x,y coordinate
You can specify x,y coordinate of the tab_button in the format:
"@x,y"
You can use this while using events
where the event argument (which tkinter passes)
to the function holds the value of x,y coordinate
of the mouse at which the event occurred which you can access by
event.x , event.y
and use that as tab_id
tab_id of currently selected tab
The currently selected tab is given
a special index of "current"
tab_id = "end"
The "end" can be used as
tab_id only in the case of index/insert method.
It returns the total number of tabs.
Changing tab's **options
# To change tab's **options you can do
myNotebook.tab(tab_id , **options)
# For example
myNotebook.tab(0,text="Changed Tab Text")
myNotebook.tab("current",text="This is current tab")
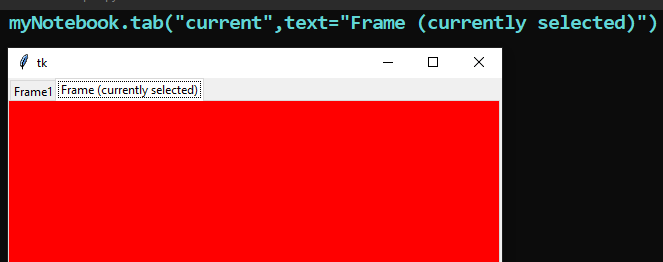
If you run this method without the **options
then tkinter will return you a dictionary
which contains options of the tab (at given index)
along with their current value.

#You can also pass the key whose value you need by the following command:
myNotebook.tab(tab_id,"text") # returns the value of text
Removing a tab
myNotebook.forget(tab_id)
# Removes the tab .
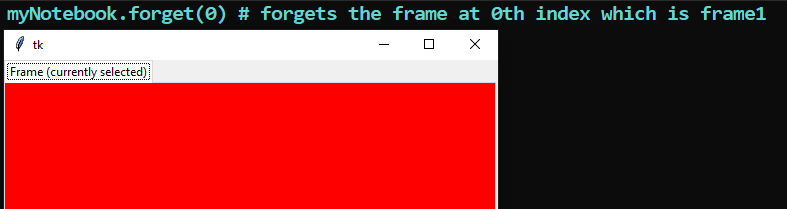
Hiding a tab button
myNotebook.hide(tab_id)
'''
Hides the given tab button.
Note: that the widget is not
removed and is still handled by
Notebook widget.
The only thing is that the button
is hidden.
'''
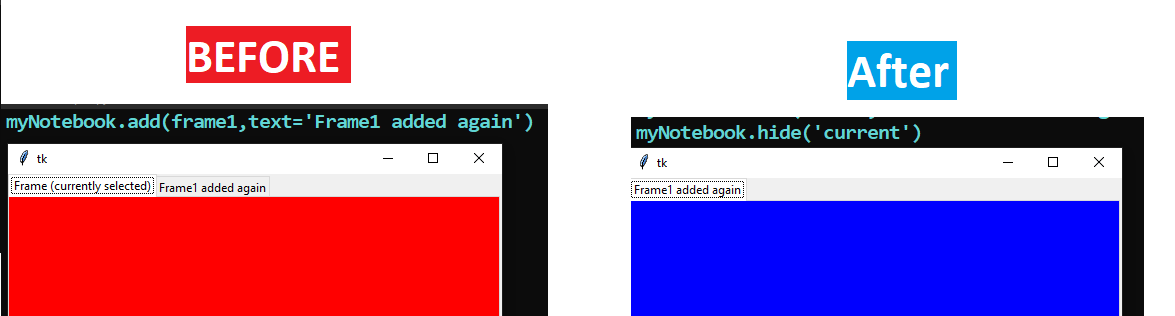
To restore the button you can use add method again.
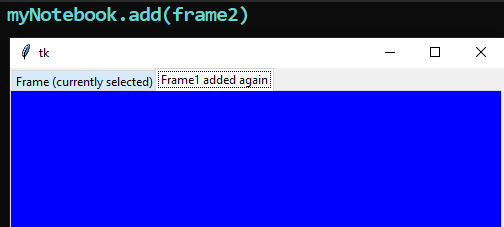
Index Method Of Notebook Widget
myNotebook.index(tab_id)
# Returns the numeric index of the tab specified by tab_id
# Returns the total number of tabs if tab_id is the string "end".
Insert Method Of Notebook Widget
myNotebook.insert(pos, tab, **options)
'''
Inserts a pane at the specified position.
pos is either the string: "end", an integer index, or the name of a new tab.
If tab is already managed by the notebook, moves it to the specified position.
'''
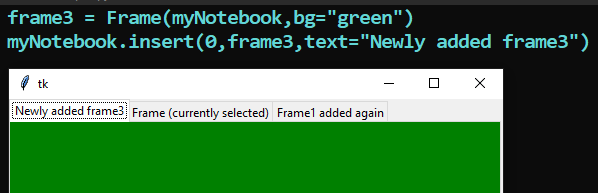
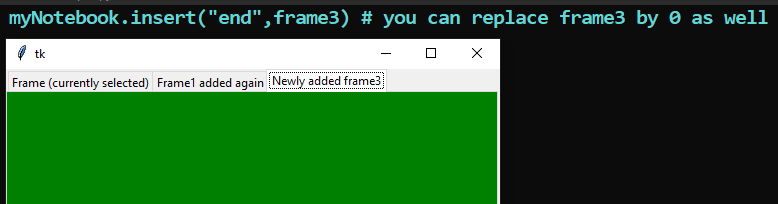
Select Method Of Notebook Widget
# To select a tab within the code and display it you can do
myNotebook.select(tab_id)
''' If tab_id is not given then the method returns the widget name of the currently selected pane '''
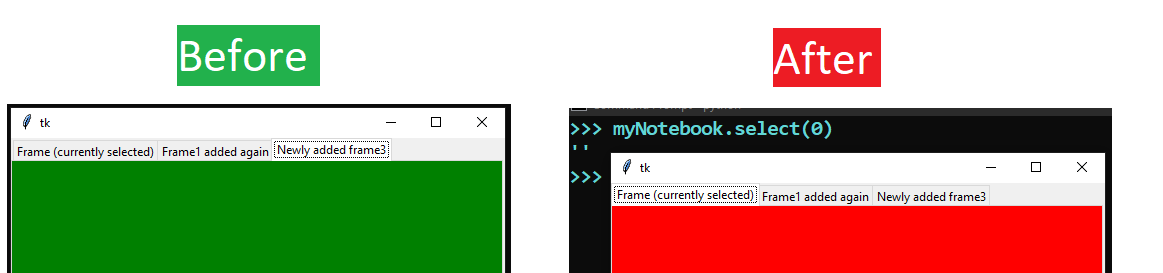
Getting all the tabs
myNotebook.tabs()
# returns all the tabs

Virtual Event : Which runs whenever user changes any tab
# You can bind the widget with the event:
"<<NotebookTabChanged>>"
Styling ttk Widgets
To style any widget you need to create an instance of
the Style class of ttk module.
myStyle = ttk.Style()
'''Now to style a ttk widget you need to get the name
of the class of that widget , which you can do by calling'''
Widget.winfo_class()
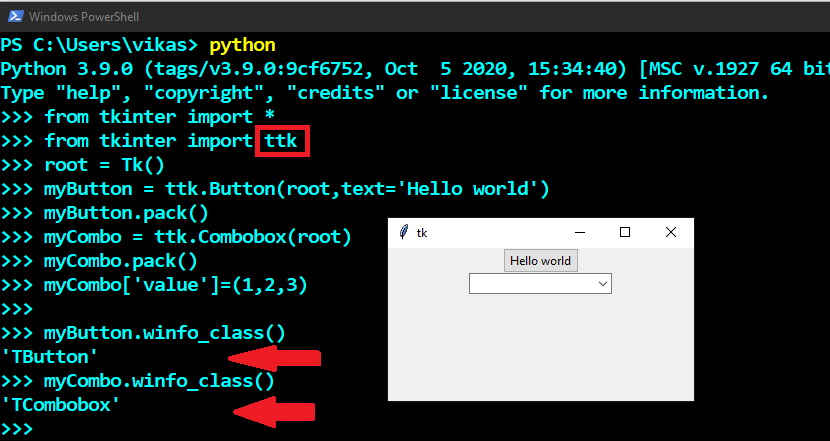
Usually the class name is : "T{NameOfWidget}" as shown above.