Python --> by myCODEnotein
Variables and Constants
Constants
Constants are fixed values which can not be changed
For ex: 1 , 1.5 , "vikas"
Variables
Variables are those values which can be changed.
For ex: a=1
# a is a variable
Rules for naming Variables
- name can contain alphabets , digits or underscores
- name cannot start with digits
- name cannot be a reserved keyword
Character Set In Python
What is a character set ?
Simply,the set of all characters/symbols
which are defined in any language are called character-sets
For ex:All alphabets (a-z) are character-sets of english language
Character set used In Python
Python uses UNICODE character set
The size of character is 2 bytes
meaning that it can contain upto 216 characters or symbols
Similarly
Similarly , there is ASCII character sets
ASCII-7(7 bits) # can contain upto 27 characters or symbols
ASCII-8(8 bits) # can contain upto 28 characters or symbols
Tokens in python
# There Are 5 types of tokens in python
- Keywords
- Literals
- Identifiers
- Operators
- Punctuators
Keywords
Keywords are fixed words which are defined by python language
# To get a list of Keywords run :
help("keywords")
# List of Keywords:
keywords = ["False", "None", "True", "__peg_parser__", "and", "as", "assert", "async", "await", "break", "class", "continue", "def", "del", "elif", "else", "except", "finally", "for", "from", "global", "if", "import", "in", "is", "lambda", "nonlocal", "not", "or", "pass", "raise", "return", "try", "while", "with", "yield"]
# keywords might vary version to version (This list is obtained from python 3.9.0)
# Note:
# you can also run:
help()
# and then type keywords
Literals
# Literals are fixed values
Examples of literals:
Numerical Literals # ex: 1(int) , 2.5(float)
String Literals # ex: "amit sir","Vikas","myCODEnotein"
Boolean Literals # ex: True , False
None
#True,False and None are keywords also.
Identifiers
Identifiers are names used to identify a piece of data like variables,name of functions,name of classes
You can also say that all keywords are also Identifiers
Rules for defining vaiables= Rules for defining Identifiers
Punctuators
( ) { } [ ] ; , . \ # : = @
etc are considered as Punctuators
Operators
# Arithmetic Operators
op: + , - , * , / , // , % , **
# The operator is known as exponential operator
for ex: 2**5
is read as: 2 raise to the power 5 (25)
returns: 32
# Operator is known as floor-division operator
for ex: 9//4
returns: 2 (/ operator returns 2.25)
desc: returns largest-integer , smaller than quotient
# Operator is known as modulus operator
for ex: 3%5
returns: 3 (remainder got by dividing 3 by 5)
desc: returns remainder
Quick Functions
Dir Function
# returns a list of functions/classes in a class or module.
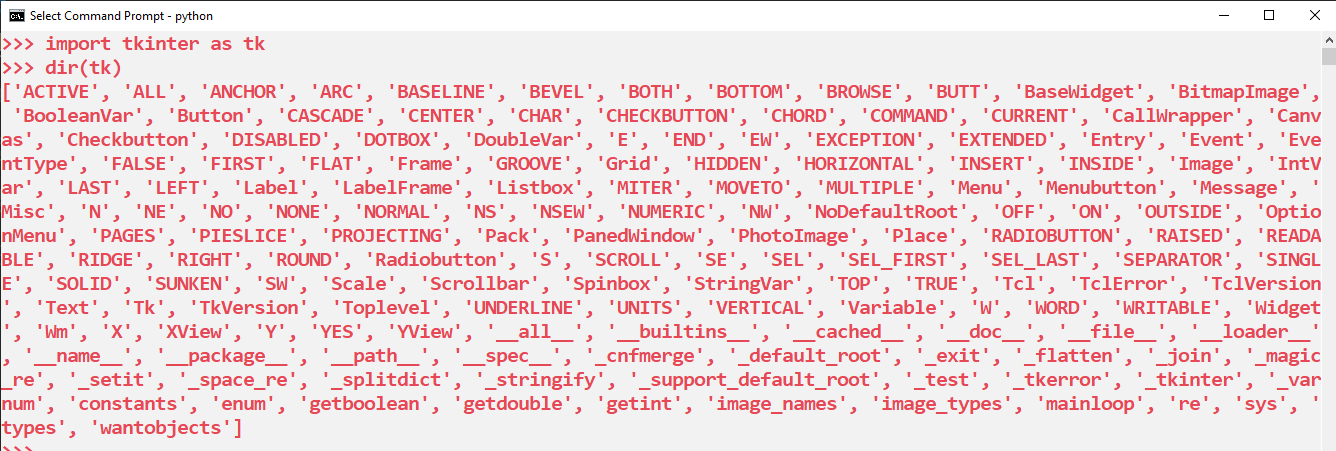
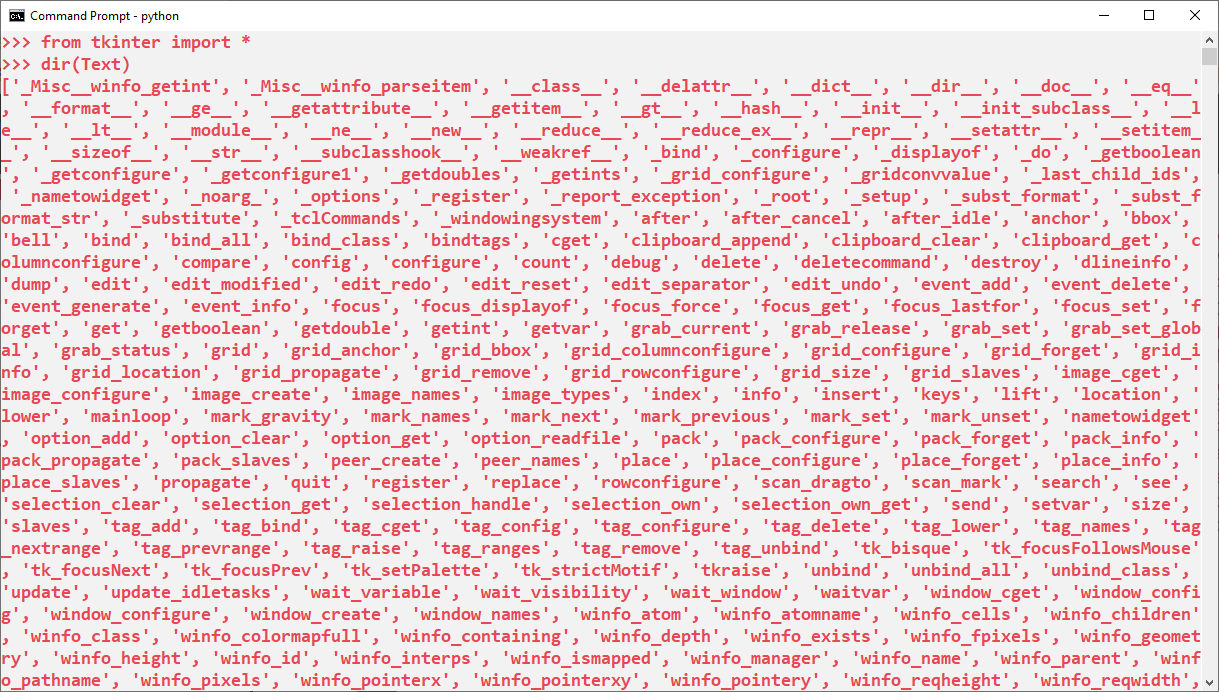
Help Function
help(argument)
# argument can be any function or class or module name
# returns a description of the given argument
Input Function
name = input("message to display")
# Used to take user input , obviously
Enumerate
# Used to get index and value while using for a loop
# Let us understand enumerate by example
for index,value in enumerate(list_of_values):
# Code Here
print(index,value)
All and Any Functions
# Let there be a list
myList = [5>0 , 6<0 , 1==0]
all(myList)==True # only if all conditions are True
# In this case all(myList) = False
any(myList)==True # even if one condition is True
# In this case any(myList) = True
Split and Strip functions
# For example:
a = "Hello world my name is vikas"
a = a.split(" ")
# Now a=["Hello","world","my","name","is","vikas"]
# For example:
a = " myCODEnotein is best "
a = a.strip()
# Now a="myCODEnotein is best"
Generators in python (yield keyword)
What are generators?
Generators are used while using functions in python.
And that function returns a list/tuple/sequence.
Generators use yield keyword.
How to create a generator?
# Example function
def myFunction(range_of_number):
for x in range(range_of_number):
# your code
yield(x)
# x can be replaced by the value which you want to return
'''
Now this function is a generator
'''
How to use a generator?
# Example function
def create_square(list_of_numbers):
for number in list_of_numbers:
yield(number*number)
# Now to use the create_square function
squared_list = create_square([1,2,3,4,5])
# First way
# You can use next function
next(squared_list) # returns sqaure of 1
'''
If you run it again it will return sqaure of 2
If you run it again it will return sqaure of 3
and so on till last element.
After that it will return Error.
'''
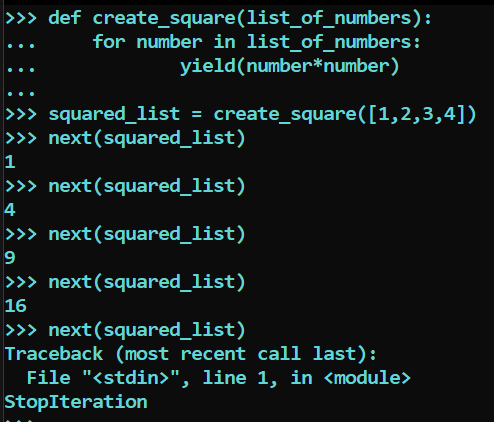
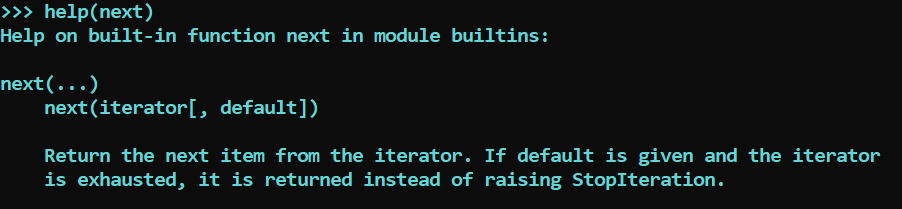
# Second way
# Using for loop
for square in squared_list:
print(square)
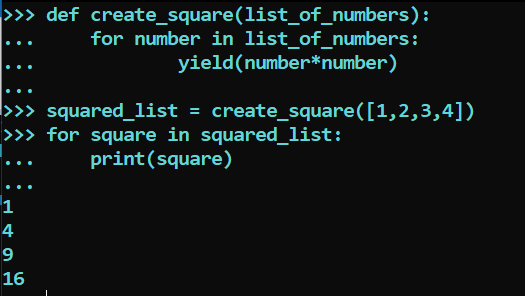
Creating a mini server with python
Just open the terminal in any folder (containing your website)
which you want to server and run the following command there:
python -m http.server port_number
# by default port_number is 8000
#For example:
python -m http.server
#(or)
python -m http.server 8000
Opening the server at a specific address
python -m http.server port_number --bind address
# For example
python -m http.server 8000 --bind 127.0.0.1
# 127.0.0.1 is by default
# and there is no need to write 8000 as well
Both IPv4 and IPv6 addresses are supported.
Opening the server in a particular directory
python -m http.server --directory path
# For example
python -m http.server --directory C://myCODEnotein
By default it will serve current working directory