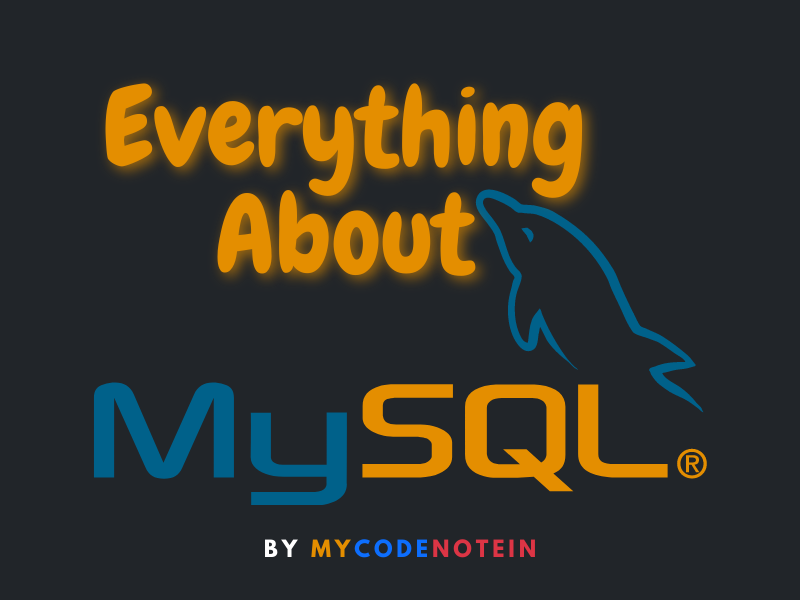
Everything About MySQL
Running mysql from cmd
mysql -u<username> -p
For ex:
mysql -uroot -p
Creating the database
CREATE DATABASE hospital ;
Let us connect with the database
CONNECT/USE hospital ;
Creating a table
CREATE TABLE name_of_table(col1 datatype constraints , ....);
CREATE TABLE patient
(sno INT PRIMARY KEY
,name CHAR(20)
,age INT
,dept ENUM ('surgery','ent','medicine','orthopaedic')
,dateOfAdm DATE
,charges INT NOT NULL
,gender ENUM('M','F') DEFAULT 'M'
);
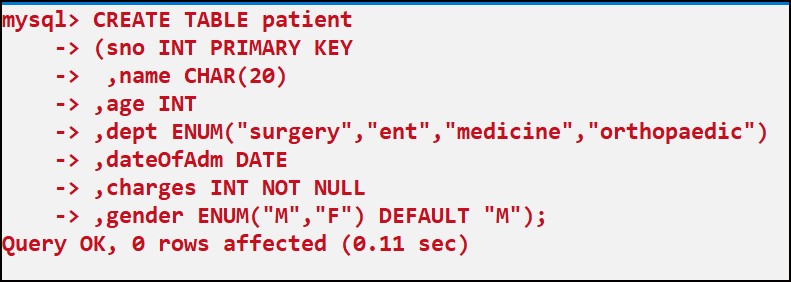
There are a lot of more constraints.
UNIQUE KEY and PRIMARY KEY constraint can be applied at row level also.
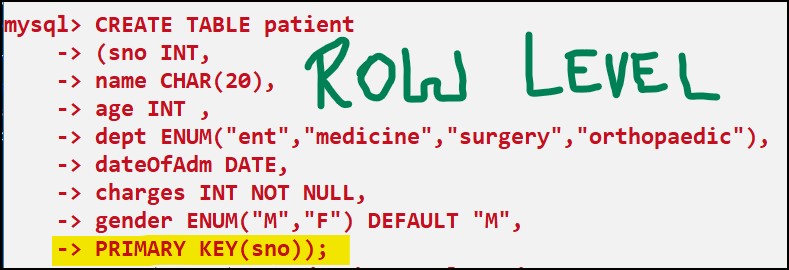
Note: you can write 'UNIQUE' in place of 'UNIQUE KEY'.
To get info about a table:
DESC/DESCRIBE/EXPLAIN nameOfTable;
Datatypes in SQL
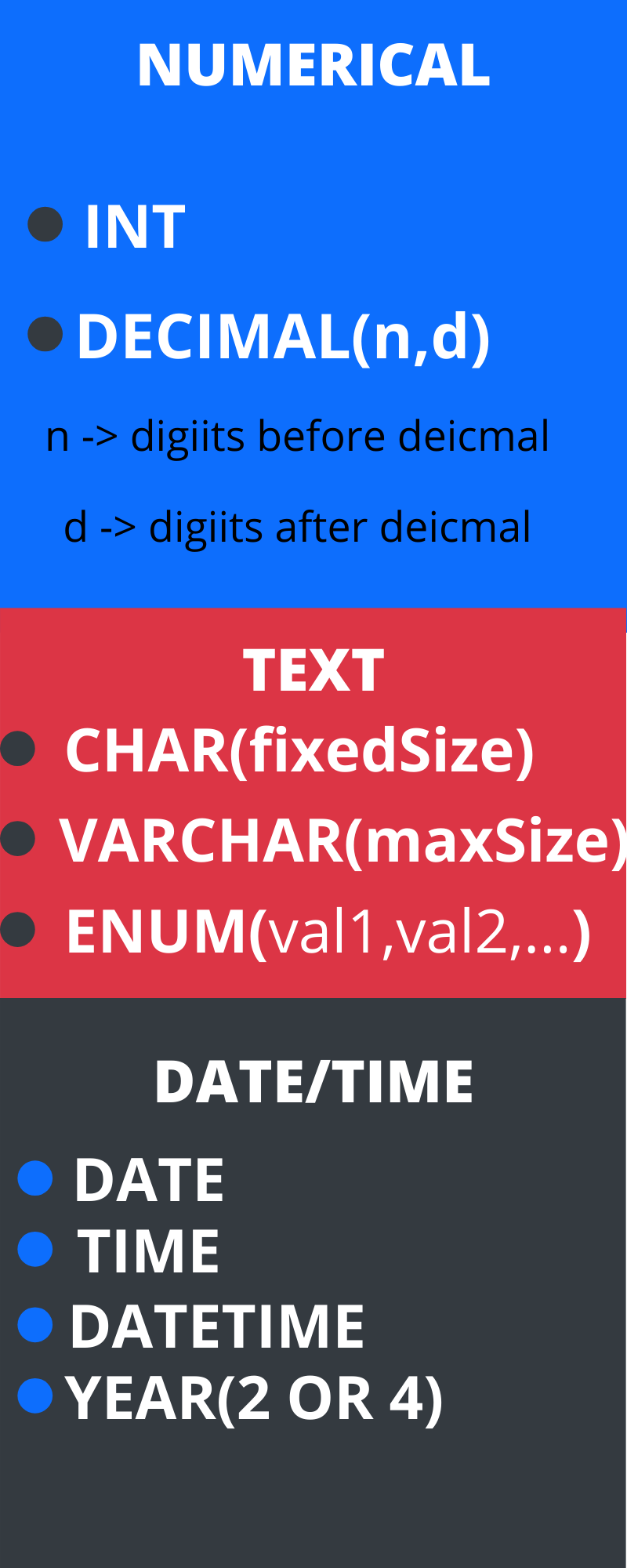
--Format of writing date,time and datetime
DATE : yyyy/mm/dd (or) yyyy-mm-dd
TIME : hh:mm:ss
DATETIME : yyyy/mm/dd hh:mm:ss
YEAR(2) -- last 2 digits of year
YEAR(4) -- all 4 digits of year
CHAR -- default size is 1 ( if not specified )
ENUM -- storage of specified values
Insert command in SQL
Insertion of one value
INSERT INTO nameOfTable VALUES (val1,val2,...valn);
You can also use DEFAULT
keyword to put the default value in column.

Insertion of one mutliple values
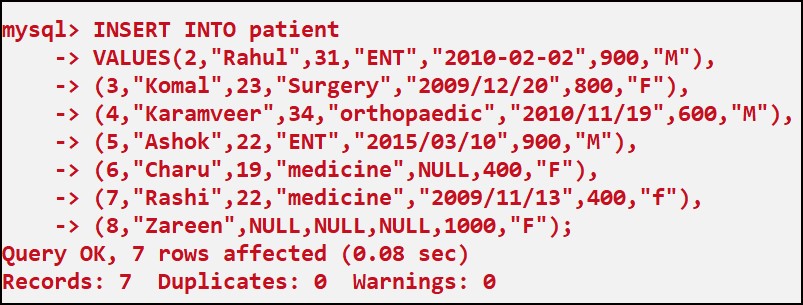
Inserting Few Columns
INSERT INTO nameOfTable
(col1,col2,...coln) VALUES (val1,val2,...valn);

Querying in SQL
SELECT columns FROM nameOfTable
WHERE conditions
ORDER BY ASC/DESC;
Note: * represents all columns
Selection of all records
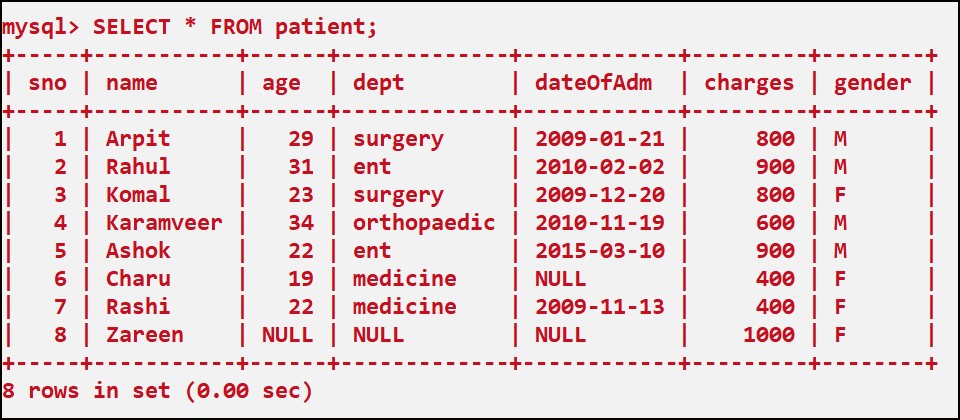
Selection of specific column
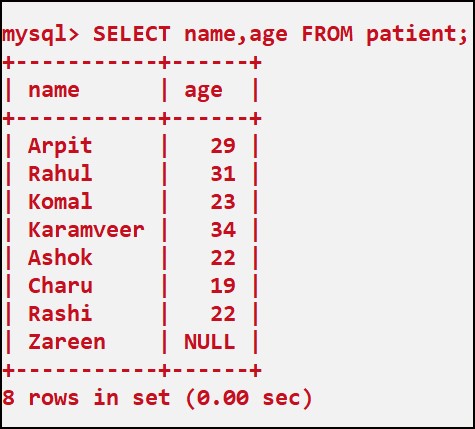
Selection of specific records
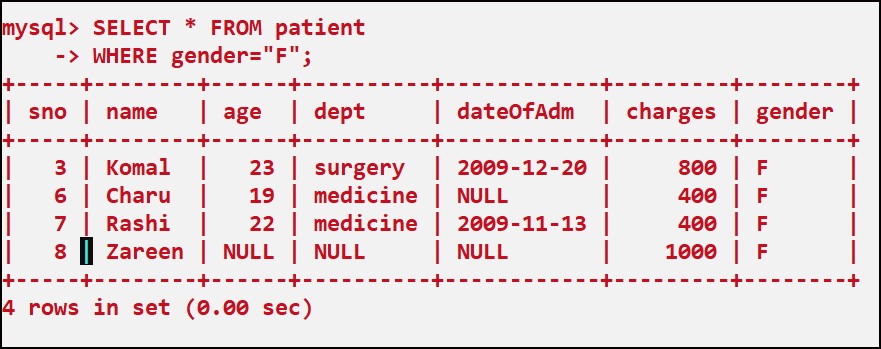
Selection of distinct/different records
DISTINCT keyword is used before column name to get distinct records.
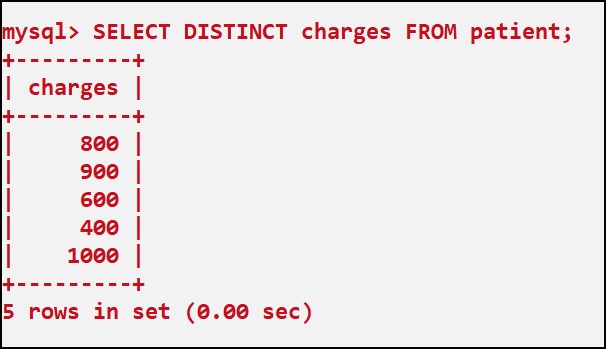
Ordering/Arranging the records
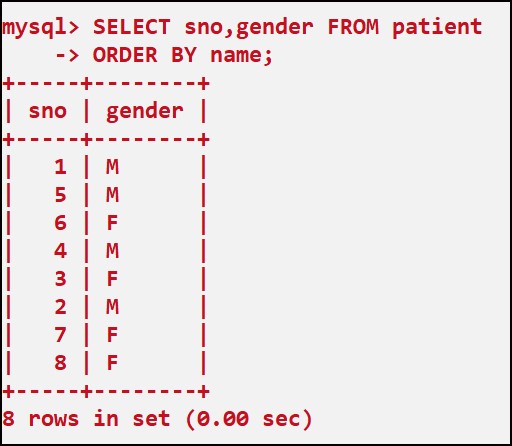
ORDER clause: ORDER BY desc/asc
default: asc
asc = ascending order
desc = descending order
If you don't write anything then by default it will show in ascending order
Displaying different name of column + expressions in SQL
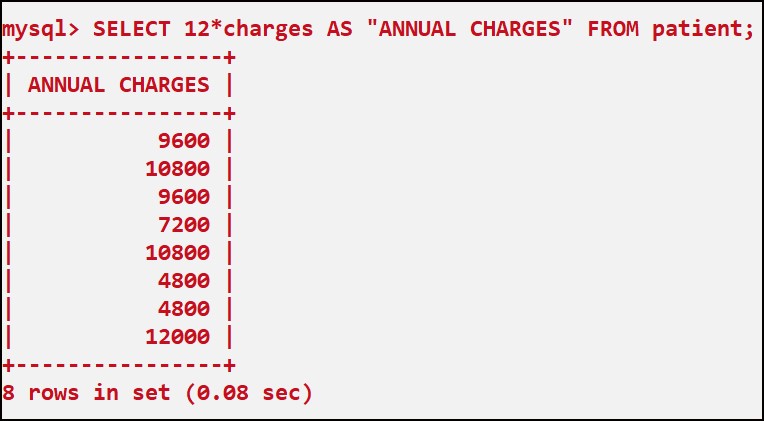
Note : AS can be used with simple columns as well
Displaying different values in records if the value is NULL
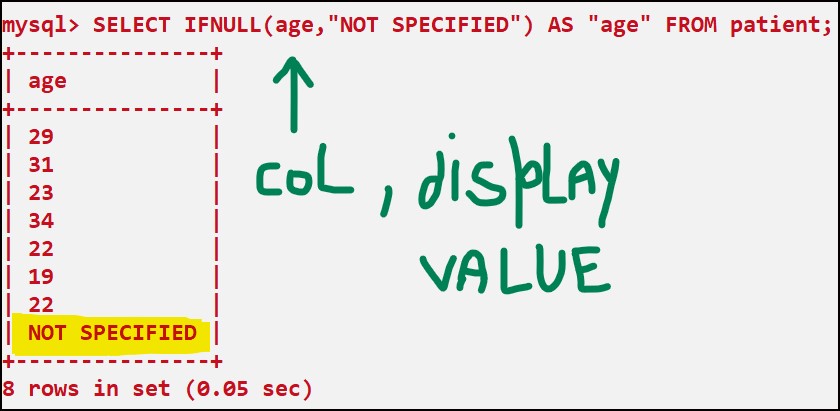
Operators in sql
Comparison operators
< , <= , > , >= , = , != or <>
Note that NULL value can not be checked by "=" operator.
It requires IS operator.
"BETWEEN" , "IN" and "IS" operator
colname BETWEEN value1 AND value2
--checks if value corresponding to the colname
--is in between value1 and value2
--or
--not
colname IN(val1,val2,...)
--checks if value corresponding to the colname
--is in the val1,val2,...
--or
--not
colname IS value
--checks if value corresponding to the colname is equal to value
To Check For null value : colname IS NULL
Note: NOT BETWEEN , NOT IN AND IS NOT ALSO EXISTS
"AND" and "OR" operator
AND can also be written as &&
condition1 AND condition2
--returns true if both conditions are true
OR can also be written as ||
condition1 OR condition2
--returns true if any condition is true
Note: NOT AND AND NOT OR DOES NOT EXISTS
Note: AND used in "BETWEEN" operator is not "AND" operator and cannot be replaced by "&&" symbol
"NOT" operator
NOT condition
Converts a condition which is true to false and vice-versa
"LIKE" operator
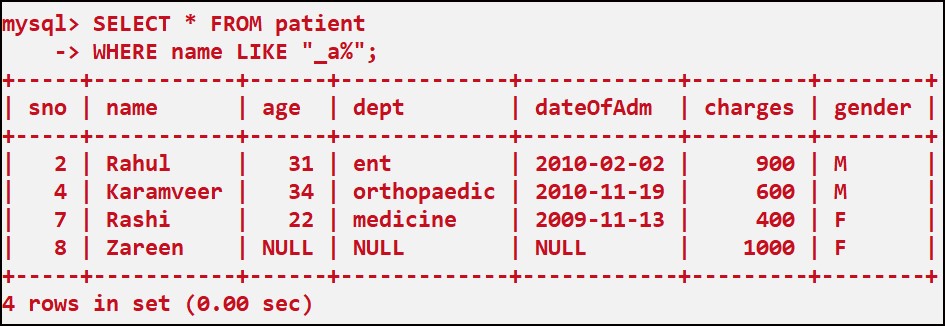
Wild Card Characters
_ REPRESENTS 1 character BEFORE "a"
% REPRESENTS any no of character(includes 0) AFTER "a"
Similarly: NOT LIKE also exists
LIKE with DATE datatype
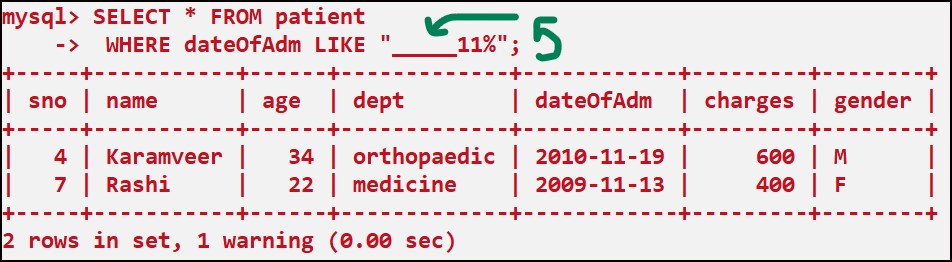
5 underscores for: yyyy/ or yyyy-
% for: another "/ or -" + any no of character in days
% is used because we don't care about no of characters after month
--Another way of doing the same thing
SELECT * FROM patient
WHERE MONTH(dateOfAdm) = 11 ;
Similarly "DAY" and "YEAR" function also exists
Update command in SQL
UPDATE nameOfTable
SET colname=value
WHERE condition;
--To change multiple columns
UPDATE nameOfTable
SET colName1 = value1 , colName2 = value2
WHERE condition;
Aggregate Functions in SQL
The aggregate functions works with multiple rows
at a time and return aggregated value.
Max/Min Function
Max function returns the maximum value of an expression.
Min function returns the minimum value of an expression.
--syntax
MAX(columname) -- for getting the max value in a column
MIN(columname) -- for getting the min value in a column
Avg function
Returns the average value of a column.
--syntax
AVG(columname)
Sum Function
Returns the sum of all the values in a column.
--syntax
SUM(columname)
Count function
Returns the count/no. of values inside a column.
--syntax
COUNT(columname) --returns count of all values inside a column
COUNT(DISTINCT columname) --returns count of distinct values inside a column
COUNT(*) -- returns count of all records in a table
Date Based Functions in SQL
Now Function
Returns the current date and time.
--Example
SELECT NOW();
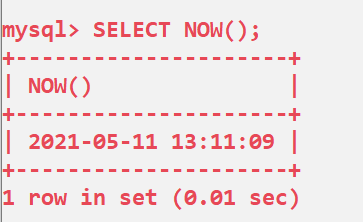
Date Function
Returns date from a string of the format
date (or) date time
--Example
SELECT DATE(NOW());
SELECT DATE('2004/07/13'); -- my birth date
SELECT DATE('2004/07/13 13:11:09');
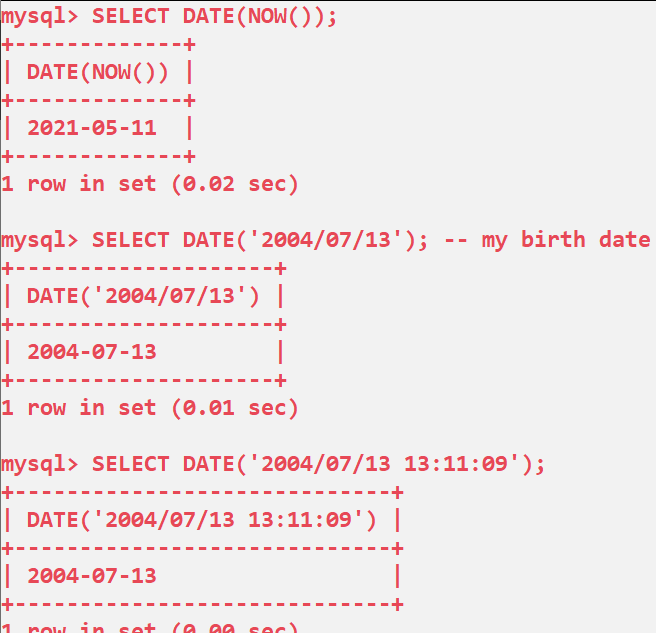
Month and MonthName Function
Month function returns month no. from date/datetime
formatted string , while monthname returns the full
name of the month.
--Example:1
SELECT MONTH(NOW());
SELECT MONTHNAME(NOW());
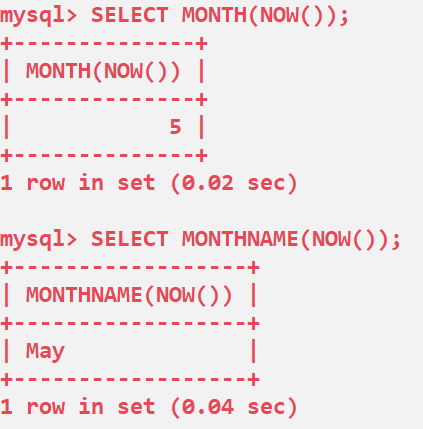
--Example:2
SELECT MONTH('2004/07/13') AS 'Birth Month No.' , MONTHNAME('2004/07/13') AS 'Birth Month Name';
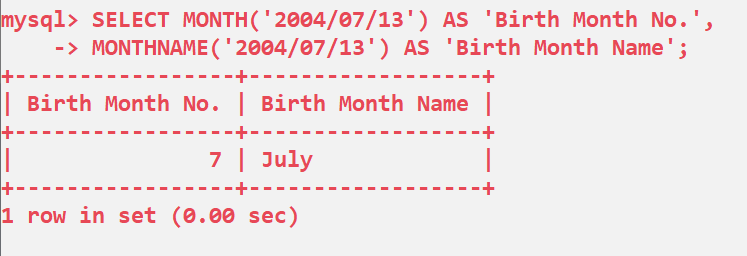
Day and Dayname function
Day function returns day no. from date/datetime
formatted string , while dayname returns the full
name of the day.
--Example:1
SELECT DAY(NOW()) , DAYNAME(NOW());
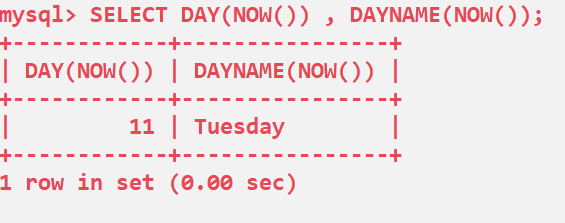
--Example:2
SELECT DAY('2004/07/13') AS 'Birth Day No.' ,
DAYNAME('2004/07/13') AS 'Birth Day Name';
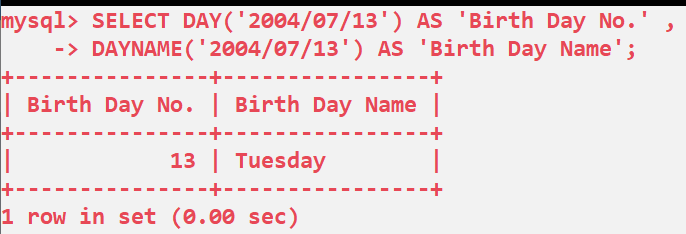
Mathematical functions in SQL
m and n (used below) can be columname : for tables
(or) numbers
(or) an algebric expression like 1+1,2*2 etc.
Power/Pow Function
returns: mn
--syntax:
POW(m,n)
--or:
POWER(m,n)
--Example
SELECT POW(2,3),POWER(3,2);
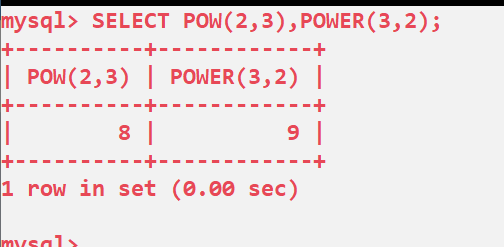
Mod Function (Remainder)
returns remainder of m/n
--syntax
m MOD n --or
MOD(m,n) --or
m % n
--Example
SELECT 2%3 , 2 MOD 3 , MOD(3,2);
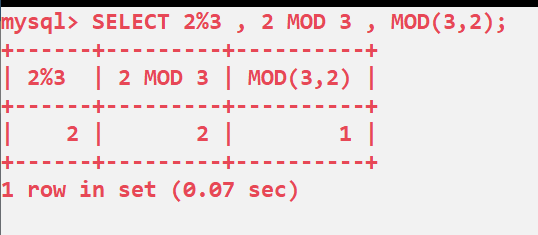
Round Function
Rounds off a number m
upto n deicmal places.
(n can be negative as well)
--syntax
ROUND(m,n)
--Example:1
SELECT ROUND(1.073,2) -- 1.07
,ROUND(1.073,1) -- 1.1
,ROUND(1.073,-1) -- 0
,ROUND(1.073,-3); -- 0
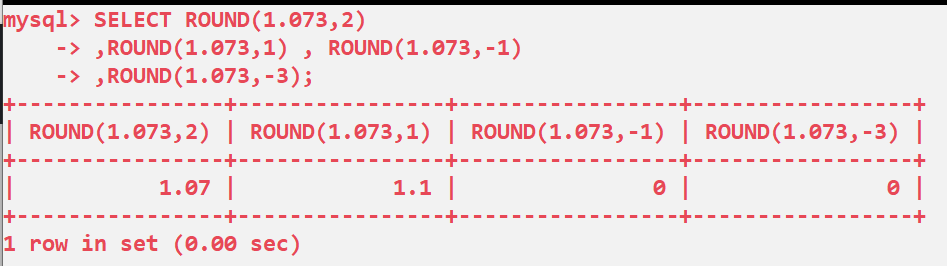
--Example:2
SELECT ROUND(149,2) -- 149
,ROUND(149,-1) -- 150
,ROUND(149,-3); -- 0
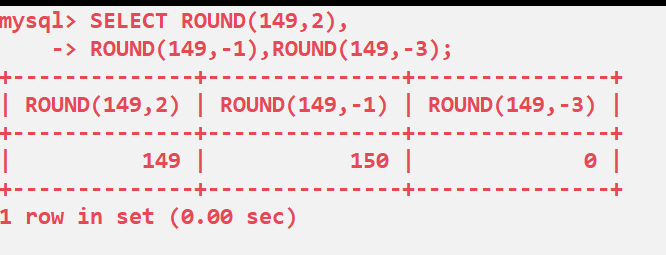
Text Based Functions in SQL
Operators like + ,- etc can be used within a function
UCASE/UPPER Function
Converts the text to uppercase.
--syntax
UCASE(string) --or
UPPER(string)
--Example
SELECT UCASE('hello') -- HELLO
,UPPER('My name is vikas!!!'); -- MY NAME IS VIKAS!!!
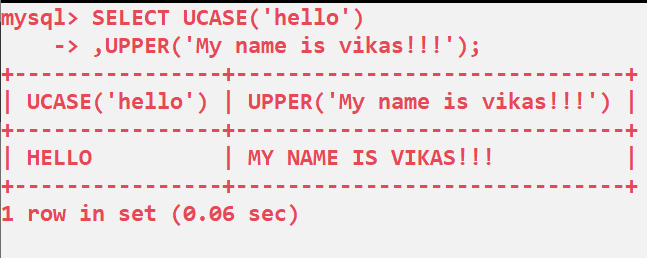
LCASE/LOWER Function
Converts the textto lowercase.
--syntax
LCASE(string) --or
LOWER(string)
--Example
SELECT LCASE('hello') -- hello
,LOWER('My name is vikas!!!'); -- my name is vikas!!!
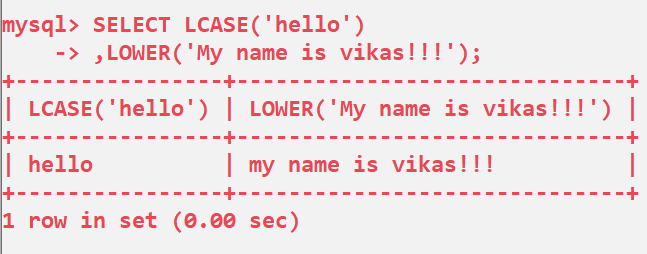
CONCAT function
CONCAT(string1 , string2 , string3 ,...)
'Used to add two strings.'
UPDATE patient
SET name = CONCAT("Mr." , name)
WHERE gender = "M" ;
LENGTH function
LENGTH(string)
'returns length of a string'
LENGTH('hello')
returns: 5
LEFT function
--Used to get starting n characters from a string
syntax : LEFT(string , n_Characters)
UPDATE patient
SET name=LEFT(name,3);
'All the name will be having first 3 characters stored only .'
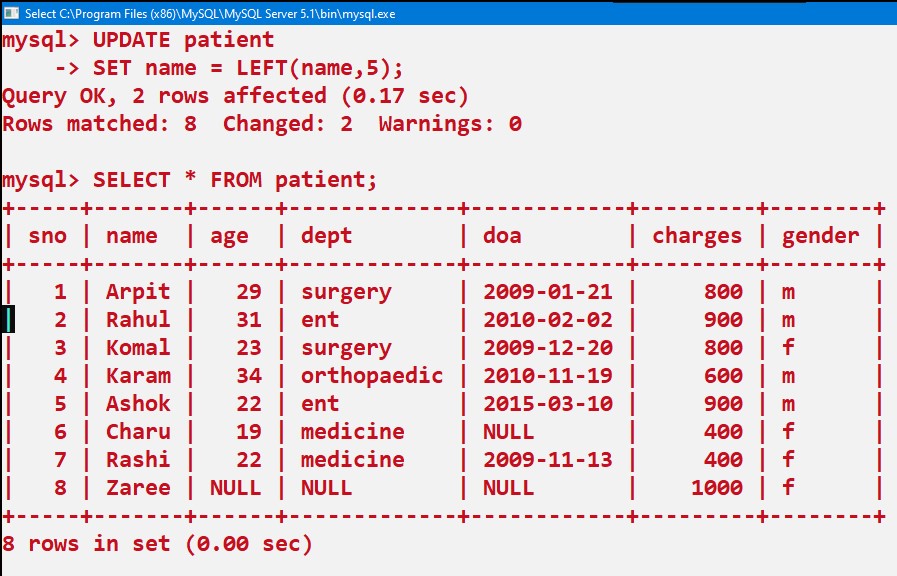
NOTE : This cannot be done on columns having datatypes like ENUM
RIGHT function
--Used to get ending n characters from a string
syntax : LEFT(string , n_Characters)
Much more advanced example of functions
'Suppose we had stored below paths in a column named file
of a table named path.
C://testpath//pygame.html
C://testpath//tkinter.html
C://testpath//tkinter-widegts.html
If we want to store only name
(pygame.html,tkinter.html,tkinter-widgets.html)
and remove the starting "C://testpath//"
then we can do that by the given code'
UPDATE path
SET file = RIGHT(file, LENGTH(file)-14)
'Here 14 is the length of C://testpath//
LENGTH(file) = LENGTH of the path
Hence , we are saying that calculate the total length of path
and then subtract 14 from it.
This will give us the no of characters
after C://testpath//
and then we can use RIGHT function
to get those file names and save them'
MID/SUBSTRING/SUBSTR function
The function is used to get a substring from a given string.
--syntax
MID(string,start_position,length)
SUBSTR(string,start_position,length)
SUBSTRING(string,start_position,length)
Position in a string starts from 1.
also you can use -ve positions.
Last character is -1 , second last is -2 and so on.
--Examples
SELECT SUBSTRING('I love my India.',2,4); -- lov
SELECT SUBSTRING('I love my India.',-2,4); -- a.
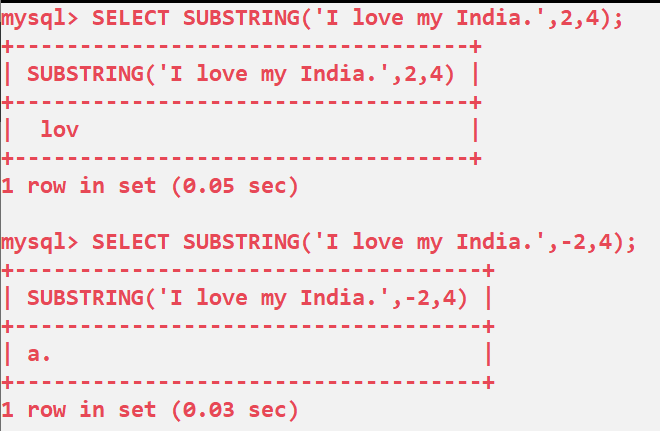
INSTR Function
Used to check if the given substring is present
in a string and returns the location of the first
character (of the substring) if the substring is present.
Positions in a string starts from 1.
If the substring is not present it returns 0.
--syntax
INSTR(string,substring)
--Example
SELECT INSTR('I Love My India','India') -- 11
,INSTR('I Love My India','Hate'); -- 0
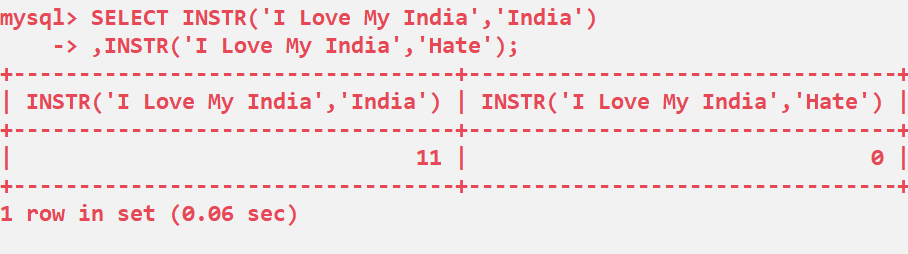
LTRIM and RTRIM Function
LTRIM function removes spaces in the starting of a string.
RTRIM function removes spaces in the ending of a string.
--syntax
LTRIM(string)
RTRIM(string)
--Example
SELECT LTRIM(' I am vikas') -- (I am vikas):result
,RTRIM('I love India ') -- (I love India):result
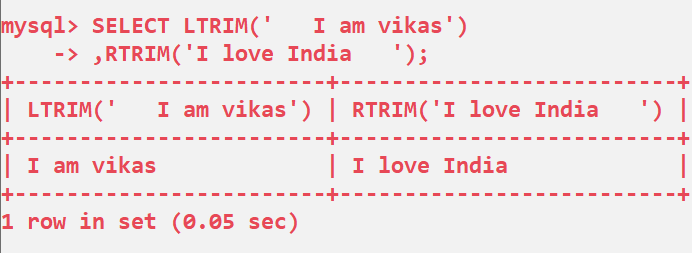
TRIM Function in SQL
Removes specified character/substring from
the specified position(Leading/Trailing/Both).
--syntax
TRIM([{LEADING/TRAILING/BOTH} {char/substring} FROM] string)
Leading : trims the char/substring present in the starting
Trailing : trims the char/substring present in the ending
Both : trims the char/substring present in both sides
char/substring : the character/substring to remove.
If char/substring and leading/trailing/both is not specified then it removes white spaces from both sides of the string , by default
--Example:1
SELECT TRIM(' I love India '); -- 'I love India'
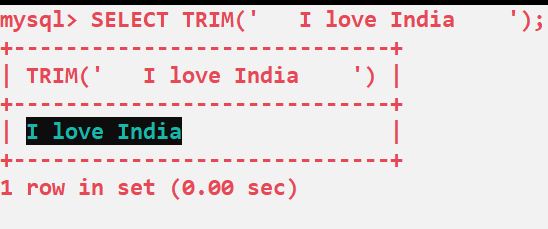
--Example:2
SELECT TRIM(BOTH 'v' FROM 'vvvI Love India vvvv')
,TRIM(LEADING 'v' FROM 'vvvI Love India vvvv')
,TRIM(TRAILING 'v' FROM 'vvvI Love India vvvv');
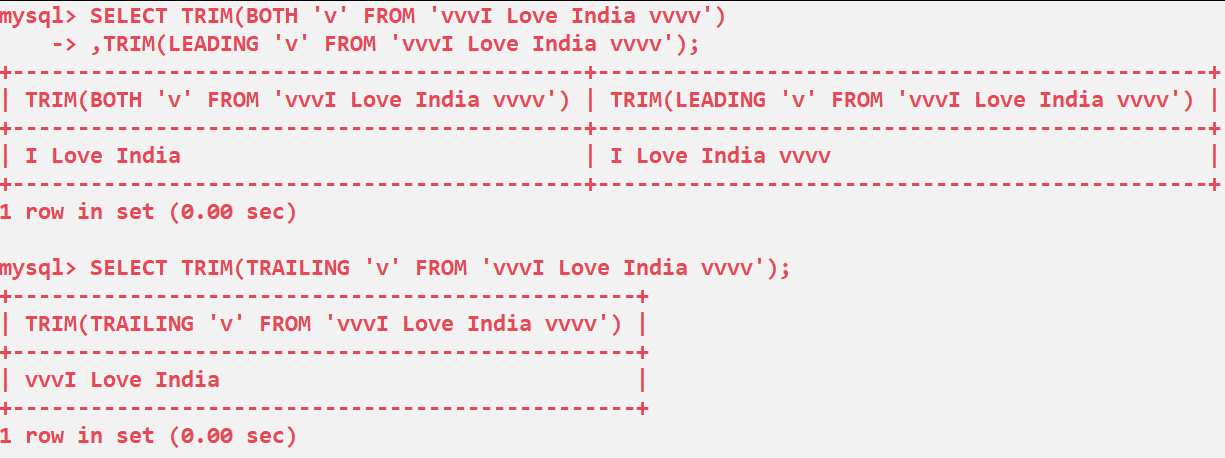
--Example:3
SELECT TRIM(LEADING 'v,' FROM 'v,v,v,vikas is my namev,v,v,');
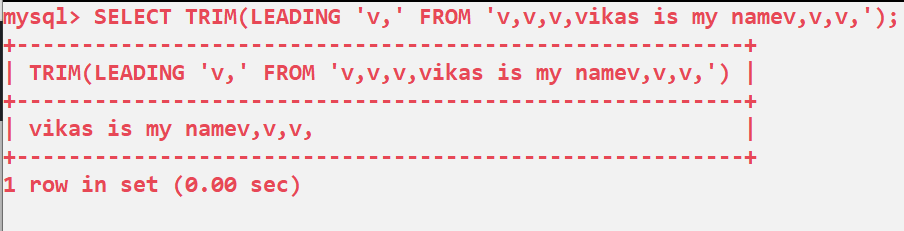
Delete command in SQL
DELETE FROM nameOfTable
WHERE condition;
Drop command in SQL
DROP DATABASE/TABLE nameOf_Datbase/Table;
Alter command in sql
ALTER TABLE nameOfTable CLAUSE changeInStructure ;
--5 clauses used :
ADD,RENAME,CHANGE,DROP,MODIFY
NOTE: Add the syntax of all clauses (EXPLAINED BELOW) after
"ALTER TABLE nameOfTable"
rename clause
--Used to rename a column
syntax: RENAME newName
Drop clause
Used to remove a COLUMN/PRIMARY-KEY/UNIQUE-KEY
--1. TO REMOVE A COLUMN:-
DROP nameOfColumn
--2. TO REMOVE THE PRIMARY KEY:-
DROP PRIMARY KEY
--3. TO REMOVE THE UNIQUE KEY:-
DROP INDEX indexOfUniqueKey
--Note: Usually indexOfUniqueKey is the name of column
--To see the index : SHOW CREATE TABLE nameOfTable;
SHOW CREATE DATABASE nameOfDatabase ;
--This is also a valid command.
Add Clause
--1. ADDING COLUMN:-
ADD colname DATATYPE CONSTRAINTS
--2. ADDING PRIMARY KEY/UNIQUE KEY :-
ADD PRIMARY KEY(nameOfCol1,nameOfCol2,..)
ADD UNIQUE KEY(nameOfCol1,nameOfCol2,..)
Change Clause
--To change/rename the column names
CHANGE oldName newName DATATYPE
Modify clause
Used to apply default,not null,null(store null value) constraint.
To change the datatype or datatype size , to reorder the columns.
--To apply constraints or change datatype or reorder:
MODIFY colName NEW/OLD_DATATYPE
NULL/NOT NULL/DEFAULT value FIRST/AFTER colname
Note: Reorder can be done in ADD clause as well
Note: applying constraint is not neccessary but if not applied then previous NOT NULL/DEFAULT constraint will be removed if exists